K means Clustering
K-means Clustering
k-means 算法源于信号处理中的一种矢量量化方法,现在则更多地作为一种聚类分析方法流行于数据挖掘领域。k-means 聚类的目的是:把 n 个点(可以是样本的一次观察或一个实例)划分到 k 个聚类中,使得每个点都属于离他最近的均值(此即聚类中心)对应的聚类,以之作为聚类的标准。详细介绍可以查看维基百科的解释
核心算法
已知观测集\((x_{1},x_{2},...,x_{n})\),其中每个观测都是一个 d-维实矢量,k-means 聚类要把这 n 个观测划分到 k 个集合中(k≤n),使得组内平方和最小。换句话说,它的目标是找到使得满足下式 \[\arg\min_S \sum_{i=1}^{k}{ \sum_{x\in S_i}{\|x_i - \mu_i \|^2} }\]
其中\(\mu_i\)是\(S_i\)中所有点的平均值
算法流程描述
下面用伪代码来描述下算法的具体过程
- 初始化 均值 \(m_1, m_2, \ldots , m_k\)
- until 均值不再改变
- 使用估计的均值根据距离最近的原则把各个样本分到相应的聚类当中
- For i from 1 to k
- 用聚类\(i\)中所有样本的均值来代替\(m_i\)
- end_for
- end_until
代码实现
下面看看 k-mean 算法具体是如何实现的,下面这段代码是生成数据的。
1 | import numpy as np |
生成的数据如下图
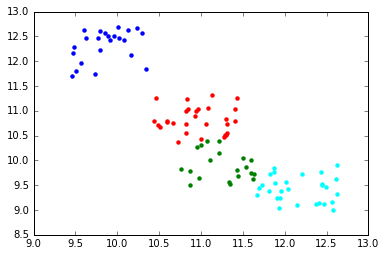
接下来的这段代码是 K 均值的核心部分
1 | from sklearn.cluster import KMeans |
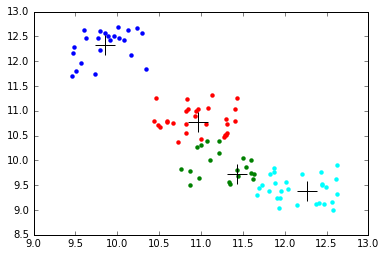